How to Decrypt and Remove Password Protection from a PDF in PHP
Unlock password-protected PDF documents with a quick, easy API call.
In a previous blog post, we walked through the process of encrypting and password-protecting PDF documents using a free API in PHP. In this tutorial, we’ll handle the reverse process - decrypting the PDF and unlocking it with the password - using another API.
Altogether, we’ll end up with a simple, efficient workflow for securing and unlocking our PDF documents programmatically. This will help streamline how our applications handle PDF document security when files enter and leave our network.
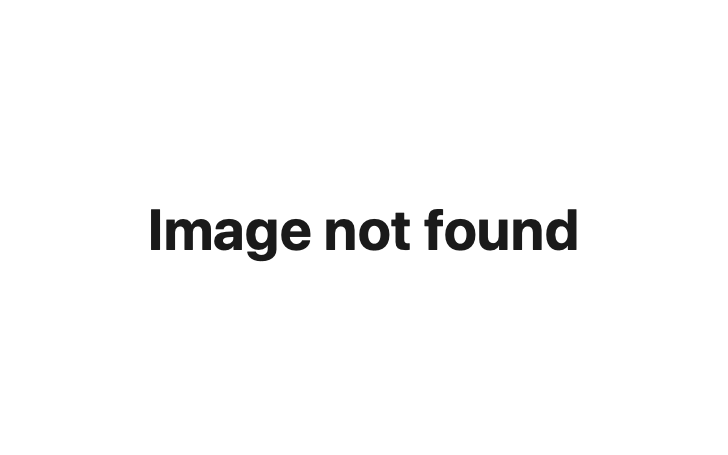
Step 1: Install the SDK
If you followed along with our encryption & password-protection tutorial, you’ll find that this process is nearly identical!
We’ll start by installing the Cloudmersive Document Convert API client with composer. Let’s run the following command:
composer require cloudmersive/cloudmersive_document_convert_api_client
Step 2: Configuration
Next, we’ll add the following configuration snippet to our file, and we’ll provide our API key to authorize our requests:
<?php
require_once(__DIR__ . '/vendor/autoload.php');
// Configure API key authorization: Apikey
$config = Swagger\Client\Configuration::getDefaultConfiguration()->setApiKey('Apikey', 'YOUR_API_KEY');
If you have an API key already, you can simply copy that from your Cloudmersive account, paste it within the ‘YOUR_API_KEY’
string, and you’re all set!
If you don’t have an API key yet, you can get one by visiting the Cloudmersive website and creating a free account. Free accounts provide a limit of 800 API calls per month with zero commitments; that total will reset each month in perpetuity. If you see the need to scale up your project, you can always review account scaling options from there.
Step 3: Instance the API
We’ll now finish up by creating an instance of the API and calling the decryption & password authentication function. Decryption is handled automatically of course, but we’ll need to supply the PDF password via $password
in our request:
$apiInstance = new Swagger\Client\Api\EditPdfApi(
new GuzzleHttp\Client(),
$config
);
$password = "password_example"; // string | Valid password for the PDF file
$input_file = "/path/to/inputfile"; // \SplFileObject | Input file to perform the operation on.
try {
$result = $apiInstance->editPdfDecrypt($password, $input_file);
print_r($result);
} catch (Exception $e) {
echo 'Exception when calling EditPdfApi->editPdfDecrypt: ', $e->getMessage(), PHP_EOL;
}
?>
In the API response, we’ll receive the encoding for a decrypted and unlocked version of our PDF document. That leaves the original secure version of the document intact, unless we choose to write the new file bytes over the original version.
Just like that, we’re all done - no more code required! If you have any questions about using Cloudmersive APIs to automate PDF document operations, feel free to reach out to any member of our team.